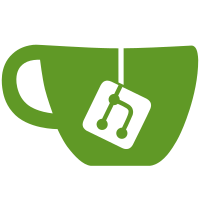
removed unused functions, removed unused variables, reduced dynamic stack memory (there was too much allocated), moved some code around into better place, implemented destructor (there is still a std::_face_node left on the stack when the program is done),
578 lines
16 KiB
C++
578 lines
16 KiB
C++
#include "Object.h"
|
|
#include <iostream>
|
|
#define PI (4.0*atan(1.0))
|
|
|
|
|
|
/////////////////////////////////////////////////////////////////////////
|
|
// public constructor/destructor
|
|
|
|
Object::Object(const char* path)
|
|
{
|
|
// open file
|
|
fsMesh.open(path, std::ios::in | std::ios::binary);
|
|
|
|
if (!fsMesh.is_open())
|
|
throw std::invalid_argument(std::string("file not found: ") += path);
|
|
|
|
// jump to file size information
|
|
fsMesh.seekg(4);
|
|
|
|
std::uint32_t tempFileSize;
|
|
std::list<ChunkHeader*> tempMainChunks;
|
|
|
|
// get all chunks under HEDR
|
|
fsMesh.read(reinterpret_cast<char*>(&tempFileSize), sizeof(tempFileSize));
|
|
loadChunks(tempMainChunks, fsMesh.tellg(), tempFileSize);
|
|
|
|
// evaluate HEDR subchunks (= find MSH2)
|
|
for (std::list<ChunkHeader*>::iterator it = tempMainChunks.begin(); it != tempMainChunks.end(); it++)
|
|
{
|
|
if (!strcmp("MSH2", (*it)->name))
|
|
{
|
|
// get all subchunks
|
|
std::list<ChunkHeader*> tempMsh2Chunks;
|
|
loadChunks(tempMsh2Chunks, (*it)->position, (*it)->size);
|
|
|
|
// evaluate MSH2 subchunks
|
|
analyseMsh2Chunks(tempMsh2Chunks);
|
|
|
|
// clean up
|
|
while (!tempMsh2Chunks.empty())
|
|
{
|
|
ChunkHeader* tempCursor = tempMsh2Chunks.front();
|
|
tempMsh2Chunks.pop_front();
|
|
delete tempCursor;
|
|
}
|
|
continue;
|
|
}
|
|
}
|
|
|
|
// clean up
|
|
while (!tempMainChunks.empty())
|
|
{
|
|
ChunkHeader* tempCursor = tempMainChunks.front();
|
|
tempMainChunks.pop_front();
|
|
delete tempCursor;
|
|
}
|
|
|
|
// close file
|
|
fsMesh.close();
|
|
}
|
|
|
|
Object::~Object()
|
|
{
|
|
// clear texture list
|
|
vTextures.clear();
|
|
|
|
// clear Model list (don't delete the elements)
|
|
vModls.clear();
|
|
}
|
|
|
|
|
|
/////////////////////////////////////////////////////////////////////////
|
|
// private functions
|
|
|
|
void Object::setModlDefault(Modl * model)
|
|
{
|
|
model->name = "";
|
|
model->parent = "";
|
|
model->type = null;
|
|
model->renderFlags = -1;
|
|
model->m4x4Translation = glm::mat4(1.0f);
|
|
model->tran.scale[0] = 0;
|
|
model->tran.scale[1] = 0;
|
|
model->tran.scale[2] = 0;
|
|
model->tran.rotation[0] = 0;
|
|
model->tran.rotation[1] = 0;
|
|
model->tran.rotation[2] = 0;
|
|
model->tran.rotation[3] = 0;
|
|
model->tran.translation[0] = 0;
|
|
model->tran.translation[1] = 0;
|
|
model->tran.translation[2] = 0;
|
|
model->swci.type = -1;
|
|
model->swci.data1 = -1;
|
|
model->swci.data2 = -1;
|
|
model->swci.data3 = -1;
|
|
model->texture = "";
|
|
model->vertex = NULL;
|
|
model->uv = NULL;
|
|
model->mesh = NULL;
|
|
model->meshSize = 0;
|
|
}
|
|
|
|
void Object::loadChunks(std::list<ChunkHeader*>& destination, std::streampos start, const std::uint32_t end)
|
|
{
|
|
// jump to first chunk
|
|
fsMesh.seekg(start);
|
|
|
|
do
|
|
{
|
|
ChunkHeader* tempHeader = new ChunkHeader();
|
|
|
|
fsMesh.read(reinterpret_cast<char*>(&tempHeader->name[0]), sizeof(tempHeader->name) - 1);
|
|
fsMesh.read(reinterpret_cast<char*>(&tempHeader->size), sizeof(tempHeader->size));
|
|
tempHeader->position = fsMesh.tellg();
|
|
|
|
destination.push_back(tempHeader);
|
|
|
|
fsMesh.seekg(tempHeader->size, std::ios_base::cur);
|
|
|
|
// reached end
|
|
if (fsMesh.tellg() - start == end)
|
|
break;
|
|
|
|
// error. Maybe the size information is corrupted
|
|
if (!fsMesh.good())
|
|
{
|
|
std::cout << "WARNING: corrupted file. Trying to continue" << std::endl;
|
|
fsMesh.clear();
|
|
break;
|
|
}
|
|
|
|
} while (true);
|
|
}
|
|
|
|
void Object::analyseMsh2Chunks(std::list<ChunkHeader*>& chunkList)
|
|
{
|
|
for (std::list<ChunkHeader*>::iterator it = chunkList.begin(); it != chunkList.end(); it++)
|
|
{
|
|
if (!strcmp("MATL", (*it)->name))
|
|
{
|
|
// "useless" information how many MATD follow
|
|
fsMesh.seekg((*it)->position);
|
|
std::uint32_t tempMatdCount;
|
|
fsMesh.read(reinterpret_cast<char*>(&tempMatdCount), sizeof(std::uint32_t));
|
|
|
|
// get all MATD from MATL list
|
|
std::list<ChunkHeader*> tempMatlChunks;
|
|
loadChunks(tempMatlChunks, fsMesh.tellg(), (*it)->size - 4);
|
|
|
|
// evaluate MATL subchunks
|
|
for (std::list<ChunkHeader*>::iterator it = tempMatlChunks.begin(); it != tempMatlChunks.end(); it++)
|
|
{
|
|
// This shouldn't be anything else than MATD
|
|
if (!strcmp("MATD", (*it)->name))
|
|
{
|
|
// get all subchunks from MATD
|
|
std::list<ChunkHeader*> tempMatdChunks;
|
|
loadChunks(tempMatdChunks, (*it)->position, (*it)->size);
|
|
|
|
// analyse MATD subchunks
|
|
analyseMatdChunks(tempMatdChunks);
|
|
|
|
// clean up
|
|
while (!tempMatdChunks.empty())
|
|
{
|
|
ChunkHeader* tempCursor = tempMatdChunks.front();
|
|
tempMatdChunks.pop_front();
|
|
delete tempCursor;
|
|
}
|
|
}
|
|
}
|
|
|
|
// clean up
|
|
while (!tempMatlChunks.empty())
|
|
{
|
|
ChunkHeader* tempCursor = tempMatlChunks.front();
|
|
tempMatlChunks.pop_front();
|
|
delete tempCursor;
|
|
}
|
|
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("MODL", (*it)->name))
|
|
{
|
|
Modl* tempModl = new Modl;
|
|
setModlDefault(tempModl);
|
|
|
|
// get all subchunks
|
|
std::list<ChunkHeader*> tempChunks;
|
|
loadChunks(tempChunks, (*it)->position, (*it)->size);
|
|
|
|
// evaluate MODL subchunks
|
|
analyseModlChunks(tempModl, tempChunks);
|
|
|
|
//clean up
|
|
while (!tempChunks.empty())
|
|
{
|
|
ChunkHeader* tempCursor = tempChunks.front();
|
|
tempChunks.pop_front();
|
|
delete tempCursor;
|
|
}
|
|
|
|
// save Model data
|
|
vModls.push_back(tempModl);
|
|
|
|
continue;
|
|
}
|
|
}
|
|
}
|
|
|
|
void Object::analyseMatdChunks(std::list<ChunkHeader*>& chunkList)
|
|
{
|
|
for (std::list<ChunkHeader*>::iterator it = chunkList.begin(); it != chunkList.end(); it++)
|
|
{
|
|
//TX1D, TX2D, TX3D
|
|
if (!strcmp("TX0D", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
char* buffer = new char[(*it)->size + 1];
|
|
*buffer = { 0 };
|
|
fsMesh.read(buffer, (*it)->size);
|
|
vTextures.push_back(buffer);
|
|
delete buffer;
|
|
continue;
|
|
}
|
|
}
|
|
}
|
|
|
|
void Object::analyseModlChunks(Modl* dataDestination, std::list<ChunkHeader*>& chunkList)
|
|
{
|
|
for (std::list<ChunkHeader*>::iterator it = chunkList.begin(); it != chunkList.end(); it++)
|
|
{
|
|
if (!strcmp("MTYP", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
std::uint32_t tempType;
|
|
fsMesh.read(reinterpret_cast<char*>(&tempType), sizeof(tempType));
|
|
dataDestination->type = (Mtyp)tempType;
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("PRNT", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
char* buffer = new char[(*it)->size];
|
|
*buffer = { 0 };
|
|
fsMesh.read(buffer, (*it)->size);
|
|
dataDestination->parent = buffer;
|
|
delete buffer;
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("NAME", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
char* buffer = new char[(*it)->size];
|
|
*buffer = { 0 };
|
|
fsMesh.read(buffer, (*it)->size);
|
|
dataDestination->name = buffer;
|
|
delete buffer;
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("FLGS", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->renderFlags), sizeof(dataDestination->renderFlags));
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("TRAN", (*it)->name))
|
|
{
|
|
float tempScale[3];
|
|
float tempRotation[4];
|
|
float tempTrans[3];
|
|
|
|
fsMesh.seekg((*it)->position);
|
|
|
|
fsMesh.read(reinterpret_cast<char*>(&tempScale[0]), sizeof(float));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempScale[1]), sizeof(float));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempScale[2]), sizeof(float));
|
|
|
|
fsMesh.read(reinterpret_cast<char*>(&tempRotation[0]), sizeof(float));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempRotation[1]), sizeof(float));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempRotation[2]), sizeof(float));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempRotation[3]), sizeof(float));
|
|
|
|
//calculate x,y,z rotation
|
|
tempRotation[0] = atan2(2 * (tempRotation[0] * tempRotation[1] + tempRotation[2] * tempRotation[3]),
|
|
1 - 2 * (pow(tempRotation[1], 2) + pow(tempRotation[2], 2)));
|
|
tempRotation[1] = asin(2 * (tempRotation[0] * tempRotation[2] - tempRotation[3] * tempRotation[1]));
|
|
tempRotation[2] = atan2(2 * (tempRotation[0] * tempRotation[3] + tempRotation[1] * tempRotation[2]),
|
|
1 - 2 * (pow(tempRotation[2], 2) + pow(tempRotation[3], 2))) - PI;
|
|
|
|
fsMesh.read(reinterpret_cast<char*>(&tempTrans[0]), sizeof(float));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempTrans[1]), sizeof(float));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempTrans[2]), sizeof(float));
|
|
|
|
dataDestination->m4x4Translation = glm::scale(
|
|
dataDestination->m4x4Translation,
|
|
glm::vec3(tempScale[0], tempScale[1], tempScale[2])
|
|
);
|
|
|
|
dataDestination->m4x4Translation = glm::translate(
|
|
dataDestination->m4x4Translation,
|
|
glm::vec3(tempTrans[0], tempTrans[1], tempTrans[2])
|
|
);
|
|
|
|
dataDestination->m4x4Translation = glm::rotate(
|
|
dataDestination->m4x4Translation,
|
|
tempRotation[0],
|
|
glm::vec3(1, 0, 0)
|
|
);
|
|
|
|
dataDestination->m4x4Translation = glm::rotate(
|
|
dataDestination->m4x4Translation,
|
|
tempRotation[1],
|
|
glm::vec3(0, 1, 0)
|
|
);
|
|
|
|
dataDestination->m4x4Translation = glm::rotate(
|
|
dataDestination->m4x4Translation,
|
|
tempRotation[2],
|
|
glm::vec3(0, 0, 1)
|
|
);
|
|
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("GEOM", (*it)->name))
|
|
{
|
|
// get all subchunks
|
|
std::list<ChunkHeader*> tempGeomChunks;
|
|
loadChunks(tempGeomChunks, (*it)->position, (*it)->size);
|
|
|
|
// evaluate GEOM subchunks
|
|
analyseGeomChunks(dataDestination, tempGeomChunks);
|
|
|
|
// clean up
|
|
while (!tempGeomChunks.empty())
|
|
{
|
|
ChunkHeader* tempCursor = tempGeomChunks.front();
|
|
tempGeomChunks.pop_front();
|
|
delete tempCursor;
|
|
}
|
|
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("SWCI", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->swci.type), sizeof(dataDestination->swci.type));
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->swci.data1), sizeof(dataDestination->swci.data1));
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->swci.data2), sizeof(dataDestination->swci.data2));
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->swci.data3), sizeof(dataDestination->swci.data3));
|
|
continue;
|
|
}
|
|
}
|
|
}
|
|
|
|
void Object::analyseGeomChunks(Modl * dataDestination, std::list<ChunkHeader*>& chunkList)
|
|
{
|
|
for (std::list<ChunkHeader*>::iterator it = chunkList.begin(); it != chunkList.end(); it++)
|
|
{
|
|
if (!strcmp("SEGM", (*it)->name))
|
|
{
|
|
// get all subchunks
|
|
std::list<ChunkHeader*> tempSegmChunks;
|
|
loadChunks(tempSegmChunks, (*it)->position, (*it)->size);
|
|
|
|
// evaluate SEGM subchunks
|
|
analyseSegmChunks(dataDestination, tempSegmChunks);
|
|
|
|
// clean up
|
|
while (!tempSegmChunks.empty())
|
|
{
|
|
ChunkHeader* tempCursor = tempSegmChunks.front();
|
|
tempSegmChunks.pop_front();
|
|
delete tempCursor;
|
|
}
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("CLTH", (*it)->name))
|
|
{
|
|
// get all subchunks
|
|
std::list<ChunkHeader*> tempClthChunks;
|
|
loadChunks(tempClthChunks, (*it)->position, (*it)->size);
|
|
|
|
// evaluate CLTH subchunks
|
|
analyseClthChunks(dataDestination, tempClthChunks);
|
|
|
|
// clean up
|
|
while (!tempClthChunks.empty())
|
|
{
|
|
ChunkHeader* tempCursor = tempClthChunks.front();
|
|
tempClthChunks.pop_front();
|
|
delete tempCursor;
|
|
}
|
|
continue;
|
|
}
|
|
}
|
|
}
|
|
|
|
void Object::analyseSegmChunks(Modl * dataDestination, std::list<ChunkHeader*>& chunkList)
|
|
{
|
|
for (std::list<ChunkHeader*>::iterator it = chunkList.begin(); it != chunkList.end(); it++)
|
|
{
|
|
/*if (!strcmp("SHDW", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
/* shadow mesh geometry
|
|
|
|
long int - 4 - number of vertex positions
|
|
float[3][] - 12 each - vertex positions (XYZ)
|
|
long int - 4 - number of edges
|
|
short int[4][] - 8 each - edge the following 4 entries from one edge
|
|
> short int - 2 - vertex index of this edge, referes to the vertex list
|
|
> short int - 2 - Reference into an edge. Defines the target vertex (the local edge vertex of the referenced edge) to which the edge should be dran from the local vertex
|
|
> short int - 2 - Second reference into an edge. In all example .msh files I've seen this always refers to the same vertex as the first edge reference
|
|
> short int - 2 - MAX_VALUE of short integers (65535). Indicates the end of this edge
|
|
* /
|
|
continue;
|
|
}*/
|
|
|
|
if (!strcmp("MATI", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
std::uint32_t tempIndex;
|
|
fsMesh.read(reinterpret_cast<char*>(&tempIndex), sizeof(tempIndex));
|
|
if (vTextures.size() <= tempIndex)
|
|
{
|
|
std::cout << "warning texture index <" << tempIndex << "> unknown" << std::endl;
|
|
dataDestination->texture = "";
|
|
continue;
|
|
}
|
|
dataDestination->texture = vTextures[tempIndex];
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("POSL", (*it)->name))
|
|
{
|
|
readVertex(dataDestination, (*it)->position);
|
|
continue;
|
|
}
|
|
|
|
/*if (!strcmp("NRML", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
// List of normals
|
|
// long int - 4 - number of normal vectores stored in this list
|
|
// float[3][] - 12 each - UVW vector for each vertex
|
|
continue;
|
|
}*/
|
|
|
|
if (!strcmp("UV0L", (*it)->name))
|
|
{
|
|
readUV(dataDestination, (*it)->position);
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("STRP", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
|
|
fsMesh.seekg((*it)->position);
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->meshSize), sizeof(dataDestination->meshSize));
|
|
|
|
dataDestination->mesh = new std::uint32_t[dataDestination->meshSize];
|
|
|
|
for (unsigned int i = 0; i < dataDestination->meshSize; i += 3)
|
|
{
|
|
std::uint16_t tempValue[3];
|
|
fsMesh.read(reinterpret_cast<char*>(&tempValue[0]), sizeof(std::uint16_t));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempValue[1]), sizeof(std::uint16_t));
|
|
fsMesh.read(reinterpret_cast<char*>(&tempValue[2]), sizeof(std::uint16_t));
|
|
|
|
if (!(tempValue[0] >> 15 && tempValue[1] >> 15 && !(tempValue[2] >> 15)))
|
|
throw std::invalid_argument("invalid file. go and triangulate!");
|
|
|
|
tempValue[0] = (std::uint16_t(tempValue[0] << 1) >> 1);
|
|
tempValue[1] = (std::uint16_t(tempValue[1] << 1) >> 1);
|
|
|
|
dataDestination->mesh[i] = (std::uint32_t)tempValue[0];
|
|
dataDestination->mesh[i + 1] = (std::uint32_t)tempValue[1];
|
|
dataDestination->mesh[i + 2] = (std::uint32_t)tempValue[2];
|
|
}
|
|
|
|
continue;
|
|
}
|
|
}
|
|
}
|
|
|
|
void Object::analyseClthChunks(Modl * dataDestination, std::list<ChunkHeader*>& chunkList)
|
|
{
|
|
for (std::list<ChunkHeader*>::iterator it = chunkList.begin(); it != chunkList.end(); it++)
|
|
{
|
|
if (!strcmp("CTEX", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
char* buffer = new char[(*it)->size];
|
|
*buffer = { 0 };
|
|
fsMesh.read(buffer, (*it)->size);
|
|
dataDestination->texture = buffer;
|
|
delete buffer;
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("CPOS", (*it)->name))
|
|
{
|
|
readVertex(dataDestination, (*it)->position);
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("CUV0", (*it)->name))
|
|
{
|
|
readUV(dataDestination, (*it)->position);
|
|
continue;
|
|
}
|
|
|
|
if (!strcmp("CMSH", (*it)->name))
|
|
{
|
|
fsMesh.seekg((*it)->position);
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->meshSize), sizeof(dataDestination->meshSize));
|
|
|
|
dataDestination->mesh = new std::uint32_t[dataDestination->meshSize * 3];
|
|
|
|
for (unsigned int i = 0; i < dataDestination->meshSize; i += 3)
|
|
{
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->mesh[i]), sizeof(std::uint32_t));
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->mesh[i + 1]), sizeof(std::uint32_t));
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->mesh[i + 2]), sizeof(std::uint32_t));
|
|
}
|
|
continue;
|
|
}
|
|
}
|
|
}
|
|
|
|
void Object::readVertex(Modl* dataDestination, std::streampos position)
|
|
{
|
|
std::uint32_t tempSize;
|
|
fsMesh.seekg(position);
|
|
fsMesh.read(reinterpret_cast<char*>(&tempSize), sizeof(tempSize));
|
|
|
|
dataDestination->vertex = new float[tempSize * 3];
|
|
|
|
for (unsigned int i = 0; i < tempSize * 3; i++)
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->vertex[i]), sizeof(float));
|
|
}
|
|
|
|
void Object::readUV(Modl* dataDestination, std::streampos position)
|
|
{
|
|
std::uint32_t tempSize;
|
|
fsMesh.seekg(position);
|
|
fsMesh.read(reinterpret_cast<char*>(&tempSize), sizeof(tempSize));
|
|
|
|
dataDestination->uv = new float[tempSize * 2];
|
|
|
|
for (unsigned int i = 0; i < tempSize * 2; i++)
|
|
fsMesh.read(reinterpret_cast<char*>(&dataDestination->uv[i]), sizeof(float));
|
|
}
|
|
|
|
|
|
/////////////////////////////////////////////////////////////////////////
|
|
// public getter
|
|
|
|
std::vector<Modl*> Object::getModels() const
|
|
{
|
|
return vModls;
|
|
}
|
|
|
|
|
|
/////////////////////////////////////////////////////////////////////////
|
|
// public functions
|
|
|