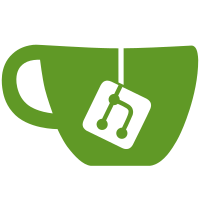
removed unused functions, removed unused variables, reduced dynamic stack memory (there was too much allocated), moved some code around into better place, implemented destructor (there is still a std::_face_node left on the stack when the program is done),
79 lines
1.6 KiB
C++
79 lines
1.6 KiB
C++
#pragma once
|
|
#include <vector>
|
|
#include <list>
|
|
#include <fstream>
|
|
#include <string>
|
|
#include <gl\glew.h>
|
|
#include <glm\gtc\matrix_transform.hpp>
|
|
|
|
enum Mtyp {
|
|
null,
|
|
dynamicMesh,
|
|
cloth,
|
|
bone,
|
|
staticMesh,
|
|
shadowMesh = 6
|
|
};
|
|
|
|
struct ChunkHeader {
|
|
char name[5];
|
|
std::uint32_t size;
|
|
std::streampos position;
|
|
};
|
|
|
|
struct Modl {
|
|
std::string name;
|
|
std::string parent;
|
|
Mtyp type;
|
|
std::uint32_t renderFlags;
|
|
glm::mat4 m4x4Translation;
|
|
struct {
|
|
float scale[3];
|
|
float rotation[4];
|
|
float translation[3];
|
|
} tran;
|
|
struct {
|
|
std::uint32_t type;
|
|
float data1;
|
|
float data2;
|
|
float data3;
|
|
} swci;
|
|
std::string texture;
|
|
float* vertex;
|
|
float* uv;
|
|
std::uint32_t* mesh;
|
|
std::uint32_t meshSize;
|
|
};
|
|
|
|
|
|
class Object
|
|
{
|
|
public:
|
|
Object(const char* path);
|
|
~Object();
|
|
|
|
private:
|
|
|
|
std::vector<Modl*> vModls;
|
|
std::fstream fsMesh;
|
|
std::vector<std::string> vTextures;
|
|
|
|
|
|
private:
|
|
void setModlDefault(Modl* model);
|
|
void loadChunks(std::list<ChunkHeader*> &destination, std::streampos start, const std::uint32_t end);
|
|
void analyseMsh2Chunks(std::list<ChunkHeader*> &chunkList);
|
|
void analyseMatdChunks(std::list<ChunkHeader*> &chunkList);
|
|
void analyseModlChunks(Modl* dataDestination, std::list<ChunkHeader*> &chunkList);
|
|
void analyseGeomChunks(Modl* dataDestination, std::list<ChunkHeader*> &chunkList);
|
|
void analyseSegmChunks(Modl* dataDestination, std::list<ChunkHeader*> &chunkList);
|
|
void analyseClthChunks(Modl* dataDestination, std::list<ChunkHeader*> &chunkList);
|
|
void readVertex(Modl* dataDestination, std::streampos position);
|
|
void readUV(Modl* dataDestination, std::streampos position);
|
|
|
|
|
|
public:
|
|
std::vector<Modl*> getModels() const;
|
|
|
|
};
|